How to request historical market data using Python
Learn how to request historical market data using Databento's Python client library with our engineering manager, Chris Sellers. Read this transcript for step-by-step instructions, or watch the video demo below to follow along in real-time.
To get started, you'll need a Databento account. Your API key is automatically generated when you sign up, and you can find it on the API keys page in your portal. Databento uses API keys to authenticate requests and for billing purposes, so make sure to keep it secure.
First, you'll need to install Databento's Python package in the command line. This library requires Python 3.8 or later.
pip install -U databento
Once installed, launch your preferred editor or terminal interface. This demo uses IPython, an interactive Python shell.
Import the databento
library and initialize the historical client to begin your data request. Replace the placeholder key with your unique API key.
import databento as db
client = db.Historical("YOUR_API_KEY")
Next, we'll use the client's timeseries.get_range
method to make a streaming request for time series data in our trades schema (data format). In this demo, we'll request the E-mini S&P 500 (ES) futures contract from February 12 to February 17, 2024, at midnight UTC.
You can find each dataset's ID in our data catalog. The ESH4
instrument trades on the CME Globex venue, so we'll specify the dataset ID for this exchange: GLBX.MDP3
.
Specify the symbol (up to 2,000 per request). We'll request the March 2024 expiring contract using its symbol, ESH4
. It's also possible to request every symbol on the venue using 'ALL_SYMBOLS'
.
In this example, we're requesting trades data. Databento's schemas (data formats) include full order book depth, aggregated order book snapshots, top-of-book quotes, bar aggregates, definitions, and more.
start
and end
) Input the data's time range. The resolution can be as granular as nanoseconds, and Databento assumes the UTC timezone unless otherwise specified. This example's time range is from Monday, February 12, to Friday, February 17, at midnight UTC (2024-02-17T00:00:00.000000000
) since a time isn't specified. The start date is inclusive, while the end date is exclusive, so the data will include messages from February 12 to February 16.
Call the timeseries.get_range
method with these parameters to download the data to your local machine via an HTTP streaming request.
import databento as db
client = db.Historical("YOUR_API_KEY")
data = client.timeseries.get_range(
dataset="GLBX.MDP3",
symbols="ESH4",
schema="trades",
start="2024-02-12"
end="2024-02-17",
)
- The
timeseries.get_range
method supports other optional parameters. - For reference, this request costs $2.17.
- The
Historical.metadata.get_cost
method can be used to determine the cost before you request any historical data. Alternatively, you can customize your request in the Databento portal for an exact quote before submitting a streaming request to the API. Read our pricing documentation for more information. - Each Databento team receives $125 in free credits for historical data, so you'll only be billed for historical requests exceeding this amount. You can see your remaining credits on the Billing page of your portal.
We can now convert the data to a pandas DataFrame using the .to_df
method, and we can see there are 1.7 million rows using Python's built-in len(df)
function.
import databento as db
client = db.Historical("YOUR_API_KEY")
data = client.timeseries.get_range(
dataset="GLBX.MDP3",
symbols="ESH4",
schema="trades"
start="2024-02-12",
end="2024-02-17",
)
df = data.to_df()
len(df)
# Returns 1735003 for this request
df.head()
Inspect the data by calling the df.head
function. By default, this method will display the first five rows of the DataFrame. For the trades schema, you'll see nanosecond-resolution receive timestamps in the leftmost column, followed by bid and ask prices, trade prices in USD, contract sizes, and the raw symbol ESH4
.
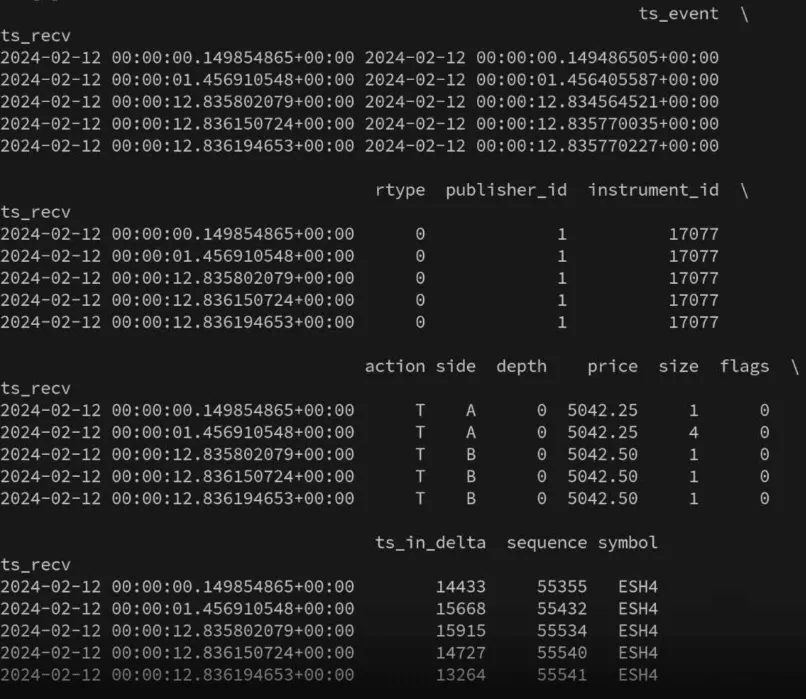
This video is part of our new API demo series, and it can be found on Databento's YouTube channel.
Our Getting started guide walks you through building your first historical or live data application with Databento. If you have additional questions, reach out to our support team.